반응형
해결 방법
맵을 이용하여 <차량 번호, {주차중인지의 여부, 입장 시간, 누적 시간}>을 map<string, bill>으로 표현했다.
bill 구조체
- 주차중인지의 여부 : 출차 기록이 나와있지 않은 경우에는 23:59에 출차되었다고 간주해야 되기 때문에 현재 주차중인지도 표시해야 한다.
- 입장 시간 : 차가 하루에 2번 이상 들어오는 경우도 있기 때문에 입장 시간을 계속 갱신해줘야 한다.
- 누적 시간 : 최종 주차 요금은 나중에 일괄적으로 계산하기 때문에 누적 시간을 반영했다.
실수할 만한 부분
- 초과 요금 계산 : 기본 시간 밑으로 주차한 경우에는 초과 요금이 0이 되어야 한다.
- 올림 연산 : 실수형 타입(float, double 등)에 대해 올림을 수행해야 한다.
ceil(excess_time/fees[2]) // X
ceil((double)excess_time/fees[2]) // O
소스 코드
#include <string>
#include <sstream>
#include <cmath>
#include <vector>
#include <map>
using namespace std;
struct bill {
bool is_using; // 현재 주차장을 사용중인가?
string enter_time; // 입장 시간
int acc_time; // 누적 시간
};
map<string, bill> m;
map<string, bill>::iterator iter;
// "mm:ss"을 mm*60+ss로 변환
int time_converter(string time) {
return stoi(time.substr(0, 2))*60+stoi(time.substr(3, 2));
}
vector<int> solution(vector<int> fees, vector<string> records) {
vector<int> answer;
for(int i=0;i<records.size();i++){
stringstream ss(records[i]);
string time, car, state;
ss >> time >> car >> state;
if(m.count(car)==0) m[car]={true, time, 0};
if(state=="IN") {
m[car].is_using=true;
m[car].enter_time=time;
} else {
m[car].is_using=false;
m[car].acc_time+=(time_converter(time)-time_converter(m[car].enter_time));
}
}
for(iter=m.begin();iter!=m.end();iter++) {
if(iter->second.is_using) iter->second.acc_time+=(time_converter("23:59")-time_converter(iter->second.enter_time)); // 자동 출차 처리
int excess_time = iter->second.acc_time-fees[0]>=0 ? iter->second.acc_time-fees[0] : 0;
answer.push_back(fees[1]+ceil((double)excess_time/fees[2])*fees[3]);
}
return answer;
}
테스트케이스 16개 모두 정답
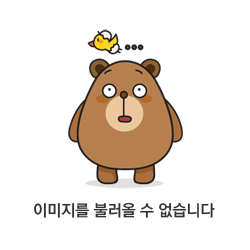
반응형
'Algorithm > 기타 알고리즘' 카테고리의 다른 글
[구간합] 파괴되지 않은 건물 - 2022 KAKAO BLIND RECRUITMENT (0) | 2022.10.04 |
---|---|
[구간합] 두 큐 합 같게 만들기 - 2022 KAKAO TECH INTERNSHIP (1) | 2022.09.30 |
[n진수] k진수에서 소수 개수 구하기 - 2022 KAKAO BLIND RECRUITMENT (0) | 2022.09.16 |
[시뮬레이션] 자물쇠와 열쇠 - 2020 KAKAO BLIND RECRUITMENT (0) | 2022.09.13 |
[시뮬레이션] 기둥과 보 (0) | 2022.09.09 |
댓글